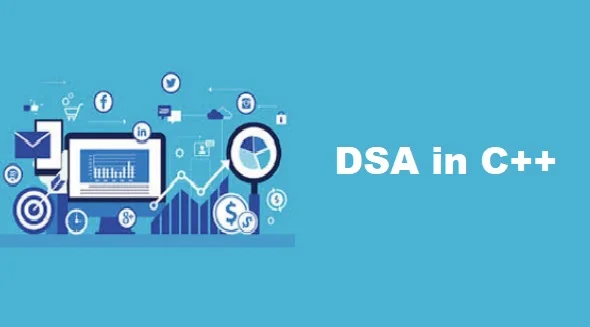
Introduction
In the world of competitive programming, mastering Data Structures and Algorithms (DSA) is akin to wielding a powerful sword in a medieval battle. It’s the cornerstone of problem-solving, enabling programmers to navigate through complex challenges efficiently. And when it comes to implementing DSA in C++, the possibilities are endless. Whether you’re a beginner stepping into the realm of competitive programming or an experienced coder looking to enhance your skills, understanding DSA in C++ is paramount.
C++ is a high-level programming language that is widely used for developing system software, application software, device drivers, embedded software, and more. It was developed by Bjarne Stroustrup in 1979 at Bell Labs as an extension of the C programming language. C++ provides a comprehensive set of features, including object-oriented programming (OOP), generic programming, and low-level memory manipulation.
One of the key features of C++ is its support for object-oriented programming paradigms, allowing developers to create classes, objects, inheritance, polymorphism, encapsulation, and abstraction. This facilitates modular and structured programming, making code organization and maintenance easier.
Additionally, C++ offers support for generic programming through templates, enabling the creation of reusable code that works with any data type. This feature promotes code efficiency and flexibility by allowing algorithms and data structures to be written once and applied to different types of data.
C++ also provides low-level programming capabilities, allowing direct manipulation of memory through features such as pointers and references. This level of control is particularly useful for tasks requiring high performance, such as system programming, game development, and embedded systems.
Moreover, C++ is known for its efficiency and performance, making it a popular choice for applications where speed and resource utilization are critical factors. Its close relationship with hardware and ability to interface directly with operating systems and hardware components contribute to its widespread use in industries such as gaming, finance, telecommunications, and real-time systems.
Getting Started: Embracing the Basics
Embarking on your journey to decode DSA in C++ starts with grasping the fundamentals. Like building a sturdy foundation for a skyscraper, a solid understanding of basic data structures and algorithms sets the stage for more intricate concepts. Let’s delve into some key data structures and algorithms:
Data Structures
- Arrays: The simplest form of data structure, arrays offer a contiguous block of memory to store elements of the same data type.
- Linked Lists: Dynamically allocated structures where each element, or node, points to the next one, forming a chain.
- Stacks and Queues: Abstract data types that adhere to Last-In-First-Out (LIFO) and First-In-First-Out (FIFO) principles, respectively.
- Trees: Hierarchical structures comprising nodes connected by edges, with a single root node at the top.
- Graphs: Non-linear data structures consisting of nodes (vertices) and edges that connect these nodes.
Algorithms
- Searching Algorithms: Techniques for finding a particular element within a data structure, such as Linear Search and Binary Search.
- Sorting Algorithms: Methods for arranging elements in a specific order, including Bubble Sort, Merge Sort, and Quick Sort.
- Graph Algorithms: Algorithms designed to traverse or manipulate graphs, such as Depth-First Search (DFS) and Breadth-First Search (BFS).
- Dynamic Programming: A method for solving complex problems by breaking them down into simpler subproblems and storing their solutions.
As you immerse yourself in understanding these basic concepts, consider enrolling in a competitive programming course. Such courses offer structured learning paths, curated materials, and hands-on practice sessions, accelerating your journey towards mastering DSA in C++.
Exploring Intermediate Concepts: Diving Deeper
Once you’ve grasped the basics, it’s time to delve deeper into intermediate concepts of DSA in C++. This phase involves understanding more complex data structures and algorithms, honing your problem-solving skills, and optimizing code efficiency. Here are some intermediate topics to explore:
Data Structures
- Binary Search Trees (BST): A specialized form of a tree where each node has at most two children, with left child nodes being lesser and right child nodes being greater. 2. Heaps: Binary trees that satisfy the heap property, crucial for implementing priority queues and heap sort.
- Hash Tables: Data structures that store key-value pairs, offering constant-time average-case complexity for insertion, deletion, and retrieval.
Algorithms
- Greedy Algorithms: Problem-solving techniques that make locally optimal choices at each step, aiming to find the global optimum.
- Backtracking: A recursive approach to solving problems by incrementally building candidates for a solution, backtracking when a candidate is deemed invalid. 3. String Algorithms: Methods for manipulating and processing strings efficiently, including pattern matching and string manipulation.
During this phase, participating in coding competitions, solving challenging problems on platforms like Codeforces or LeetCode, and collaborating with peers can significantly enhance your understanding and proficiency in DSA in C++.
Mastering Advanced Techniques: Reaching the Pinnacle
As you advance on your journey, mastering advanced techniques becomes paramount to stand out in the competitive programming arena. This phase involves delving into cutting-edge data structures, sophisticated algorithms, and optimization strategies. Here are some advanced topics to conquer:
Data Structures
- Fenwick Trees (Binary Indexed Trees): Efficient data structures for range queries and updates, particularly useful in scenarios involving cumulative frequency or sum calculations. 2. Segment Trees: Versatile structures for handling range queries and updates over an array or another linear data structure.
Algorithms
- Dynamic Programming Optimization: Techniques like memoization, state compression, and divide and conquer optimization to optimize dynamic programming solutions. 2. Advanced Graph Algorithms: Algorithms for solving complex graph problems, such as Dijkstra’s algorithm for single-source shortest paths and Floyd-Warshall algorithm for all-pairs shortest paths.
In addition to diving deeper into these advanced topics, actively participating in hackathons, contributing to open-source projects, and engaging in research endeavors can elevate your expertise in DSA in C++.
Conclusion
In conclusion, mastering DSA in C++ is a journey filled with challenges, discoveries, and triumphs. From understanding the basics to conquering advanced techniques, every step on this path enriches your problem-solving skills and computational thinking abilities. By enrolling in a competitive programming course, embracing a growth mindset, and actively engaging in practice and collaboration, you can decode the intricacies of DSA in C++ and emerge as a formidable contender in the world of competitive programming. So, embark on this exhilarating journey, and unlock the limitless potential of DSA in C++ to shape a brighter future in the realm of technology and innovation.